Introduction to Django
What is a framework?
A programming framework is a collection of pre-written code that provides a foundation for developing applications. It offers tools, libraries, and guidelines to streamline development and reduce repetitive tasks. Frameworks help maintain structure, improve efficiency, and ensure consistency in code.
Use Case:For example, when building an e-commerce site with Django, it handles tasks like authentication, URL routing, etc. Its built-in admin panel and ORM simplify managing data and orders. Without a framework, you'd have to build all this functionality from scratch.
Frontend Frameworks: React, Vue.js, Angular, etc.
Backend Frameworks: Django, Flask, Express, etc.
Overview of Django Framework
Django is a web framework written in Python that helps developers build websites quickly and easily. It provides tools for handling common tasks like database management, user authentication, and URL routing. Django is designed to make web development faster, more secure, and more efficient.
- Django follows MVT (Model-View-Template) architecture.
- Popular sites using Django: Instagram, Pinterest, Disqus, YouTube, and Spotify.
Official Site: https://www.djangoproject.com
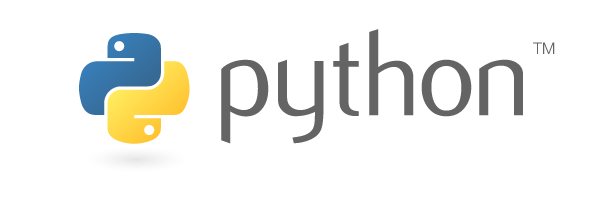
MVT vs MVC Architecture?
Quick Installation Guide
Install Python:To use Django, you need to install Python, as it is a Python-based framework. Python includes a built-in lightweight database called SQLite, so you don't need to set up a separate database initially. You can download the latest version of Python from python.org and verify the installation by typing python in your terminal.
python --version
# Completely Remove Existing Python from Your System
# Step 1: Uninstall Python from Control Panel
# Step 2: Remove Python paths from Environment Variables
# Step 3: Delete leftover folders from C:\Users\< YourUser >\AppData\Local\Programs\Python
To install Django, use pip, Python's official package manager. Run the following command in your terminal:
# Install Django using pip
python -m pip install Django
# Check Django version using django-admin
django-admin --version
# Check using Python module
python -m django --version
# Verify the version using Python's interactive shell
>>> import django
>>> django.get_version()
'5.1.5'
Exercise
- Fill in the Blanks:
- - Django is a __________ written in Python that simplifies web development.
- - Django follows the __________ architecture, which stands for __________.
- - To install Django, the package manager used is __________.
- - You can check the installed Django version using the command __________.
- - MVC stands for __________.